How to Add Conditional Content to the Top of WooCommerce Cart and Checkout Pages – by Product Category
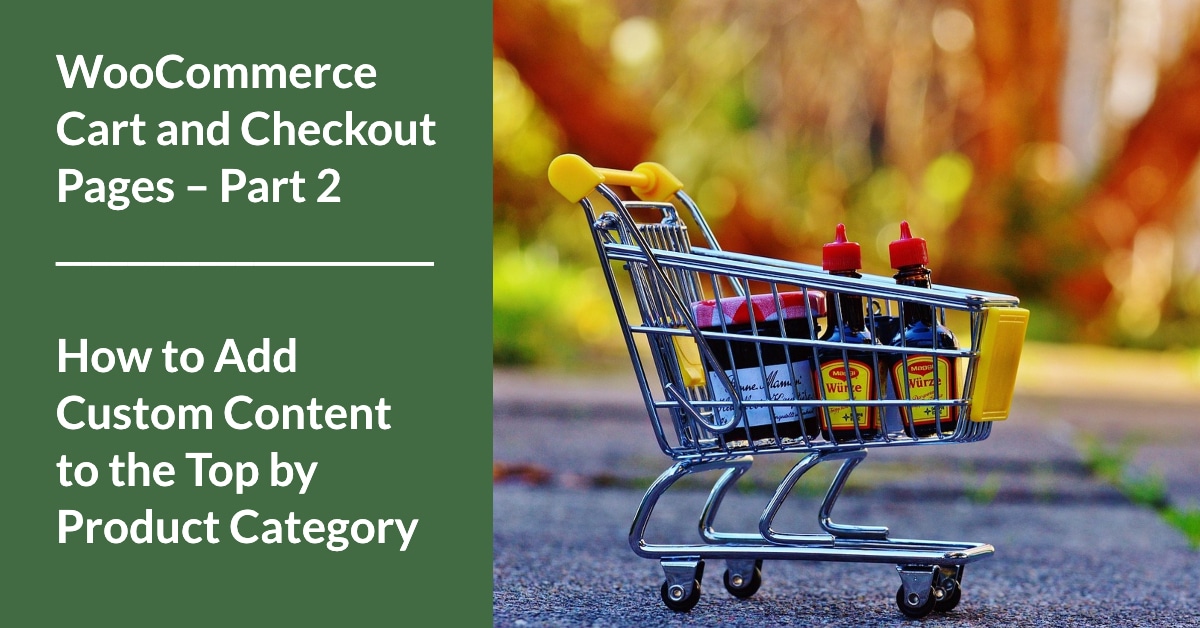
In part 2 of our WooCommerce Cart and Checkout Pages series, we’re going to take our hook from part 1 and make the function conditional based on which Product Categories are currently in the cart.
Let’s get moo-vin’!
>> Missed part 1 and want to see how we created our initial function? Catch up here!
///
Why would you need to add conditional content by Product Category?
Adding content to the cart and checkout pages is super handy. And believe it or not, one of the more common requests we get is to only show a message to customers who have certain products in their carts.
For example, we had a client that sold medications for livestock. Since those products require a prescription, we needed to warn customers about that requirement.
- While we were at it, we also gave them the opportunity (with a pop-up form) to upload their prescriptions right there on the checkout page.
- Of course, if you’re not purchasing a prescription, there is certainly no need for you to see that warning.
///
How do you add conditional content by Product Category?
To illustrate how to do this, we’re going to add (to our existing function from part 1) a conditional statement for the above prescription med scenario.
In the video demo (link below), I’m using the standard demo content from WooCommerce. You’ll see that we’re going to make our function conditionally display only if any of the products in the cart have a category of “music.”
—
Step 1 – Create a loop function.
- We need to create a function that will loop through all of the items (or “objects” in PHP-land) in the customer’s cart.
- Then we need to create a list (or “array”) of all the categories that show up.
—
Step 2 – Compare the array intersect.
Then we need to compare the array we just built with the category (or categories) that we’re looking for. (This is called an “array intersect.”)
What this function will do is evaluate two different arrays.
- If any of the items in “array 1” match any of the items in “array 2,” the function will return “true.”
- We’ll then use that “return” of true or false (a boolean) to base our conditional statement on.
- If there’s a match in the two arrays, then the function will show our awesome content.
- If not, do nothing.
///
Let’s take a look at the code!
Firstly, I can’t take credit for this code, but I can’t give credit either because I’ve forgotten where I found it! Just like most of us, I checked a hundred different Stack Overflow pages before finding what I wanted.
Secondly, this code is very nicely commented, so I highly recommend reading all the blue comments below.
I like to show the code visually since VSCode (Visual Studio Code) looks so much better than just plain text.
If you click on the image above, it will bring you to the gist where you can copy it, if you’d like. Or you can view the cart/checkout code from the video.
—
What’s the code doing, in a nutshell?
As with many PHP functions, there are multiple ways of doing this. I have just found that this one makes sense to me and continues to work quite well.
- In a nutshell, this function is getting all the items in the cart (line 9).
- Then it creates an array of all the categories in the cart (line 18) by looping through each one and pulling just the category “slug.”
- Pro tip: This function would work just as nicely if we extracted the category “ID” or “NAME.” You would just need to adjust your array in the code below to reflect that change.
- Lastly (line 22), it will return “true” if the “array intersect” we talked about above finds a match.
—
How is $categories being defined?
You will notice that the two arrays it is comparing (line 22) are defined on line 3 and line 18, but how is $categories being defined?
In order for this function to work correctly, there needs to be a value assigned to the $categories variable. This may get a little in the weeds of PHP functions, but it’s super important to our conditional hook.
In a PHP function (line 3), this is called an “argument,” but it’s not like the ones you have with your siblings!
- An argument is something we’ve indicated that our function needs to properly run.
- In our case, we need that argument as the second array for our conditional on line 22 to evaluate.
—
Let’s take a look at our updated hooks!
As the title of this post indicates, we’re now going to make sure our Cart has a specific category (or categories) in it before we display our awesome content.
As I mentioned just above this image, we need to define our variable “$categories” and then pass that into the function we created up above.
- On line 6, we create an array and place the desired product category into it.
- In this case, we’re looking for the category “slug” of “music.”
- If we want to check for multiple categories, we can just add more like this: array(‘music’, ‘cows’, ‘chickens’). You just need to separate them with commas.
- On line 7, we call up the function we created above and pass the argument of $categories into it.
- So now, when the function runs, it will use our variable of $categories as the argument in the array_intersect on line 22 in the first image of the code.
Again we can read this as:
- If there is a product with the category of “music” in our shopping cart, echo our content.
- If not, do nothing.
///
Watch the training video ► How to Add Conditional Content to the Top of WooCommerce Cart and Checkout Pages – by Product Category
In case you’re more of a watch-er than a read-er, I put all the stuff above into the video below!
—
Coming up next: What if you only want to show your custom function if there are certain products in the cart? We’ll cover that in part 3 of WooCommerce Cart and Checkout Pages series!
Want to only show your custom function if there are certain Product IDs in the cart?
Check out part 3 of our series!
. . .